threejs-3D动画
threejs-3D动画
用threejs库实现加载3d模型的动画:
点击:在线预览
主要代码:
<!DOCTYPE html> <html> <head> <title>技术藤-3D动画</title> <link type="text/css" rel="stylesheet" href="./css/main.css"> </head> <body> <div id="info" style="color:#2DC3E8;font-size:20px;margin-top:30px;text-align: right;padding-right: 40px;"> <a href="https://www.jishuteng.com">Threejs-3D动画</a> </div> <script async src="https://unpkg.com/es-module-shims@1.3.6/dist/es-module-shims.js"></script> <script type="importmap"> { "imports": { "three": "./js/three.module.js", "three/addons/": "./js/" } } </script> <script type="module"> import * as THREE from 'three'; import Stats from 'three/addons/libs/stats.module.js'; import { OrbitControls } from 'three/addons/controls/OrbitControls.js'; import { GLTFLoader } from 'three/addons/loaders/GLTFLoader.js'; let stats, mixer, camera, scene, renderer, clock; init(); animate(); function init() { const container = document.createElement( 'div' ); document.body.appendChild( container ); camera = new THREE.PerspectiveCamera( 30, window.innerWidth / window.innerHeight, 0.001, 1000 ); camera.position.set( 20, 6, 12 ); scene = new THREE.Scene(); scene.background = new THREE.Color( 0xCBCCCE ); scene.fog = new THREE.Fog( 0xCBCCCE, 70, 100 ); clock = new THREE.Clock(); const geometry = new THREE.PlaneGeometry( 500, 500 ); const material = new THREE.MeshPhongMaterial( { color: 0xCBCCCE, depthWrite: false } ); const ground = new THREE.Mesh( geometry, material ); ground.position.set( 0, - 5, 0 ); ground.rotation.x = - Math.PI / 2; ground.receiveShadow = true; scene.add( ground ); const grid = new THREE.GridHelper( 500, 100, 0x000000, 0x000000 ); grid.position.y = - 5; grid.material.opacity = 0.2; grid.material.transparent = true; scene.add( grid ); const hemiLight = new THREE.HemisphereLight( 0xffffff, 0xeeeeee, 0.6 ); hemiLight.position.set( 0, 100, 0 ); scene.add( hemiLight ); const dirLight = new THREE.DirectionalLight( 0xffffff, 0.5 ); dirLight.position.set( 0, 20, 10 ); dirLight.castShadow = true; dirLight.shadow.camera.top = 18; dirLight.shadow.camera.bottom = - 10; dirLight.shadow.camera.left = - 12; dirLight.shadow.camera.right = 12; scene.add( dirLight ); const loader = new GLTFLoader(); loader.load( './model/scene.gltf', function ( gltf ) { scene.add( gltf.scene ); gltf.scene.traverse( function ( child ) { if ( child.isMesh ) { child.frustumCulled = true; child.castShadow = true; child.material.emissive = child.material.color; child.material.emissiveMap = child.material.map ; } } ); mixer = new THREE.AnimationMixer( gltf.scene ); mixer.clipAction( gltf.animations[ 0 ] ).play(); } ); renderer = new THREE.WebGLRenderer({ antialias:true, alpha:true }); renderer.setPixelRatio( window.devicePixelRatio ); renderer.setSize( window.innerWidth, window.innerHeight ); //renderer.shadowMap.enabled = true; container.appendChild( renderer.domElement ); stats = new Stats(); container.appendChild( stats.dom ); const controls = new OrbitControls( camera, renderer.domElement ); controls.enablePan = false; controls.minDistance = 5; controls.maxDistance = 50; } function animate() { requestAnimationFrame( animate ); if ( mixer ) mixer.update( clock.getDelta() ); render(); stats.update(); } function render() { renderer.render( scene, camera ); } </script> </body> </html>
扫描二维码在线预览
除特别注明外,本站所有文章均为技术藤原创,转载请注明出处来自https://www.jishuteng.com/article/29.html
分享到微信朋友圈
扫一扫,手机阅读
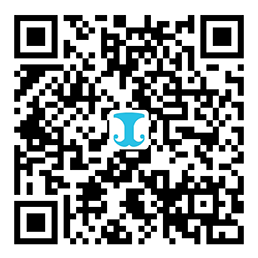
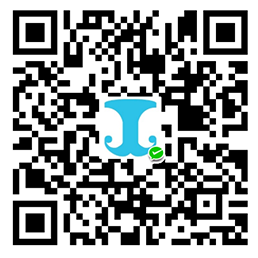
参与评论 0条评论